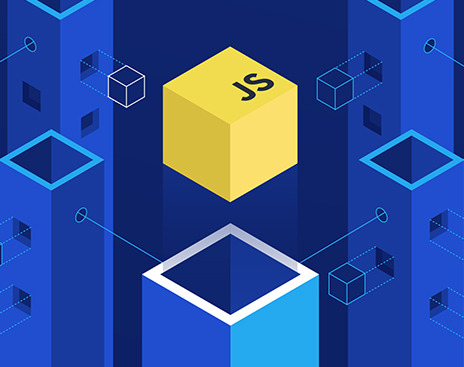
getAbsoluteUrl
Getting an absolute URL from a variable string is not as easy as you think. There is the URL constructor but it can act up if you don’t provide the required arguments (which sometimes you can’t). Here’s a smooth trick for getting an absolute URL from and string input:
var getAbsoluteUrl = (function() {
var a;
return function(url) {
if(!a) a = document.createElement(‘a’);
a.href = url;
return a.href;
};
})();
// Usage
getAbsoluteUrl(‘/something’); // https://demosite.com/something
The “burn” element href handles and URLs for you, providing a reliable absolute URL in return.
once
There is time when you prefer a given functionality only happen once, similar to the way you would use an onload event. This code provides you said functionality:
function once(fn, context) {
var result;
return function() {
if(fn) {
result = fn.apply(context || this, arguments);
fn = null;
}
return result;
};
}
// Usage
var canOnlyFireOnce = once(function() {
console.log(‘Fired!’);
});
canOnlyFireOnce(); // “Fired!”
canOnlyFireOnce(); // nada
The once function ensures that a given function can only be called once, thus prevent duplicate initialization as well.