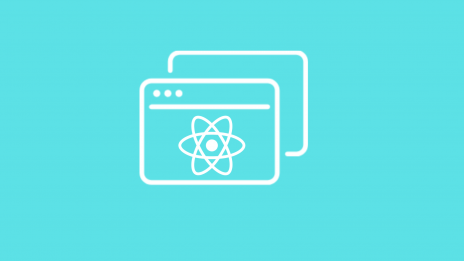
React is One of the most popular front end libraries used nowadays. React is an open-source front end library for building user interface which is mainly used by single-page applications. React was first created by Jordan Walke, a software engineer working for Facebook and it’s deployed on Facebook’s news feed in 2011 and on Instagram.com in 2012.
Features of React JS
- JSX
- Virtual Dom
- Single directional data flow
- Reusable Component
- Hooks
Reusable Component
The reusable component is the heart of the React Application. Building a reusable component will reduce the code size and you can make use of the single logic available wherever you need. There are many npm modules available which are used to write reusable component. Here we will explain how to write a reusable component using reactstrap, redux from, redux
Creating a Reusable Component
In this section, we will create a reusable component for input and a button. The first step to create react reusable component is to start with simple component which will be easy to understand for you
- Modal Popup Component
Modal Pop up Component
The modal pop up component is used to show a popup window on the current page. This component mostly used to show extra information without redirecting or change current page content. Modals are widely used to warn users for situations like session time out or to receive their final confirmation before going to perform any critical actions such as saving or deleting important data.
● Prerequisites
Believe you have already completed the react installation process. If not, please follow the steps mentioned here.
● Create Folder
Create form folder inside component folder (src > Component > Form > ModalPopup.js ) and create a modalpopup.js file inside form folder
● Importing the Required Files
We have used Hooks feature to write the components. To write the react component first we need to import react.
import React from ‘react’;
● Reusable Function
Write functional to get props and use the props to generate a reusable component
import React, {Component, useState} from ‘react’;
import { Modal , Button } from ‘react-bootstrap’
export function CustomModal(props){
- const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
return(
<div>
<Modal show={props.show} onHide={handleClose} size={props.size} centered={props.center} >
{props.header &&
<Modal.Header>
<Modal.Title>{props.title}</Modal.Title>
<button type=”button” className=”close” aria-hidden=”true” onClick={props.onClick}>×</button>
</Modal.Header>
}
<Modal.Body>{props.children}</Modal.Body>
{props.footer &&
<Modal.Footer>
{props.footercontent}
</Modal.Footer>
}
</Modal>
</div>
);
}
Note : Copy this code and past this in (src > component > modal > modalpopup.js ) modalpopup.js file
● How to use this Modal Popup Component
We have created a reusable modal popup component now we need to use this component in some pages to see how it works. To use this select component we need to create a file container folder (src > container > Pages > modalpopup.js ) and name it as modalpoup.js. Follow the steps to use this component
● Import the Component and Required Modules
- Import react
– importReact,{Component} from “react”; - Import filed and redux form
– import { Field, reduxForm, } from ‘redux-form’; - Import the component that we created
– import { select } from ‘../../../Component/Modal/ModalPopup;
● Utilizing Modal Popup Component
You can import the component where necessary and use the modal popup component as mentioned below. You can use this code with bootstrap to get a rich user interface
<div className=”popup-example”>
<Button type=”button” onClick={this.handleShow} className=”btn-primary” text=”Launch demo modal” />
<CustomModal title=”Terms & Conditions” show={this.state.execute.customModalDisplay} onClick={this.handleClose} header={true} footer={true} size={“lg”} center={true}
footercontent={<Fragment><Button type=”button” className=”btn-primary” text=”Popover” />
<Button type=”button” onClick={this.handleModalCloseButton} className=”btn-primary” text=”Close” /></Fragment>}>
Welcome
</CustomModal>
</div>
● Modal Popup Component Properties
- title
- Title of the popup will be displayed here .
- show
- When trueThe modal will show itself..
- onClick
- This callback is used to handle close modal popup
- header
- Header section of the modal popup.
- footer
- Footer content of the modal
- size
- Based on this property will be rendered like Large, Extra Large and Small. To mention you have to pass the following values.
- lg(Large)
- xl(Extra Large)
- sm
- center
- This is a callback functions this will be triggered after date has been selected.
- onShow
- This callback will be fired when popup opening.
- Based on this property will be rendered like Large, Extra Large and Small. To mention you have to pass the following values.
● Sample Class Component
We have implemented bootstrap with the date range component in below code
import React,{Component, Fragment} from “react”;
import asyncComponent from ‘../../../Router/async’;
import { Field, reduxForm, SubmissionError } from ‘redux-form’;
import { PopoverComponent } from ‘../../../Component/Popover’
import { Card } from ‘../../../Component/Card/Card’
import { Button } from ‘../../../Component/Button’
import { FormValidation } from ‘../../../validation/forms’
import { CustomModal } from ‘../../../Component/Modal/Modal’
import Tabs from ‘../../../Component/Tabs/Tabs’;
import {renderToString} from ‘react-dom/server’;
import { ProgressBarComponent } from ‘../../../Component/Progressbar’
const handleFormSubmit=(values)=>{
alert(JSON.stringify(values))
}
class ModalPopup extends Component{
constructor(props){
super(props);
this.state ={
execute:{
customModalDisplay:false,
size:”lg”,
center:true,
header:true,
title:”Terms & Conditions”,
footer:true,
footercontent:”test”
},
preview:{
customModalDisplay:false,
size:”lg”,
center:true,
header:true,
title:”Terms & Conditions”,
footer:true,
footercontent:”test”
}
}
this.hadletextboxchange = this.hadletextboxchange.bind(this)
}
componentToString(component) {
return <pre>{component}</pre>
}
coverttoString(String) {
return renderToString(String);
}
getdetails(){
return ` props: {
customModalDisplay: ${this.state.execute.customModalDisplay},
size: ${this.state.execute.size},
center: ${this.state.execute.center},
header: ${this.state.execute.header},
title: ${this.state.execute.title},
footer: ${this.state.execute.footer},
footercontent: ${this.state.execute.footercontent}
}`
}
gettabs(){
return this.componentToString(`<CustomModal customModalDisplay:”${this.state.execute.customModalDisplay}”, size=”${this.state.execute.size}”, center:”${this.state.execute.center}”, header:”${this.state.execute.header}”, title:”${this.state.execute.title}”, footer:”${this.state.execute.footer}”, footercontent:”${this.state.execute.footercontent}”></CustomModal>`)
}
hadletextboxchange = (e) =>{
let final_value = e.target.value
console.log(‘123’,final_value)
try {
final_value = JSON.parse(final_value);
this.setState({
preview:{
customModalDisplay: final_value.customModalDisplay,
size: final_value.size,
center: final_value.center,
header: final_value.header,
title: final_value.title,
footer: final_value.footer,
footercontent: final_value.footercontent
}
})
}
catch(error){
alert(error.message)
}
}
handleShow=()=>{
let modalState = this.state
modalState.execute.customModalDisplay = true;
this.setState({modalState})
}
handleClose=()=>{
let modalState = this.state
modalState.execute.customModalDisplay = false;
this.setState({modalState})
}
handleModalCloseButton=()=>{
let modalState = this.state
modalState.execute.customModalDisplay = false;
this.setState({modalState})
}
render(){
const { handleSubmit } = this.props;
return(
<div className=”row”>
<Tabs>
<div label=”Reference”>
<div className=”descriptions”>
<h2>ModalPopup Component</h2>
<hr/>
<p>Modalpopup is the fundamental component for onclick info visibility. It’s a wrapper around react-popper, that adds support for transitions, and visibility toggling.</p>
</div>
<div className=”popup-example”>
<Button type=”button” onClick={this.handleShow} className=”btn-primary” text=”Launch demo modal” />
<CustomModal title=”Terms & Conditions” show={this.state.execute.customModalDisplay} onClick={this.handleClose} header={true} footer={true} size={“lg”} center={true}
footercontent={<Fragment><Button type=”button” className=”btn-primary” text=”Popover” />
<Button type=”button” onClick={this.handleModalCloseButton} className=”btn-primary” text=”Close” /></Fragment>}>
Welcome
</CustomModal>
</div>
</div>
</Tabs>
</div>
);
}
}
export default ModalPopup
Note : Copy this code and past this in (src > Container > Pags > modalpoup.js ) modalpopup.js file
● Modal Popup Component Preview
After creating a date range class component you can run the react file and see the following output.
Installations
To write reusable component with react and redux you need to install following npm module
- npm install -g create-react-app
-g is used to install the npm module globally this will make the module and –save is used while to save the installed module in package.json file.
Once after installing create-react-app run following commands to create a react project
- Create-react-app reusable-component
The above command will create a reusable-component bundled with inbuilt npm modules such as react, react-dom. We need some more modules to create reusable components. Install the following module
- npm install –save react-redux
- npm install –save redux
- npm install –save bootstrap
After installing this module you need to import required function from the module to that make it easy to use
Folder Structure In React
There is no predefined structure in react projects. It’s your idea to format the folders. Here we mentioned the best practices for folder structure. We have split components into a container and presentational component according to the component usage which makes it easy to use.